Example
Importing and initialising pygame
As we do with any module in python we need to import pygame:
Debian - pygame is available through apt-get (1.9.1) FreeBSD - pygame is included in standard ports as py26-game (1.8.1) OpenBSD - pygame is included in standard ports (1.8.1) Macintosh These are packages for the python from python.org, not the apple provided python. Browse other questions tagged python python-3.x visual-studio pygame or ask your own question. The Overflow Blog Podcast 331: One in four visitors to Stack Overflow copies code. You can use any editor to edit your code on your computer, but you will find it very helpful to get familiar with a code editor, such as Visual Studio Code. Visual Studio Code supports both Windows and Mac and it's free. To install it, go to and follow the instructions.
We then initialise all the imported pygame modules:
This is used to initialise all of the pygame modules. Without this the modules would not work
Defining Constants
We then define some constants here:
The WIDTH
and HEIGHT
constants are used to create a window, which would have a width of 300 pixels and a height of 200 pixels. The function used in SCREEN
, pygame.display.set_mode((WIDTH, HEIGHT))
, will set the mode of the display and return a Surface object. Note how the parameters for this function are the WIDTH
and HEIGHT
constants defined earlier.
Setting the name of the window
We then use this function to change the name of the window to My Game:
Defining colours
Afterwards we define 6 colours that can be used in our window:
When defining colours we put in 3 values that range between 0 and 255. The pygame.Color class normally goes by this format:
Visual Studio Code Vs Visual Studio
Where the r parameter sets the red value of the colour, the g parameter sets the green value of the colour and the b parameter sets the blue value of the colour. The a parameter sets the alpha value of the colour.
We then give this command:
This is a pygame.Surface.fill function which fills the Surface object, our screen, with the colour red.
Using pygame.display.flip()
We then use this function
This basically makes everything we have drawn on the screen Surface become visible and updates the contents of the entire display. Without this line, the user wouldn't see anything on their pygame screen.
The game loop
The next few lines are what's called a 'game loop'.
To start this off we make a variable and make it True:
So that we can start off our while loop:
which will be running throughout the whole game.
In it's most basic form, pygame has 'events' which takes user input, for example a button press or mouse click. Pygame handles these events through an event queue. We can get these events from the event queue with this for loop:
Which basically goes through a list of events, our event queue. These are the next 2 lines:
This will make it so that when the user presses the exit button in the top corner, the event with the type pygame.QUIT
occurs.
This then ends the while loop, as is_running
is now False
and the script moves on to the final line:
Which uninitialises the pygame modules.
Not sure what to download? Read the Installation Notes.
1.9.6 Packages (April 25th 2019)
Source
- pygame-1.9.6.tar.gz ~ 3.1M ~ d923c554203a7c35e37921658cb4c5bf50215ab0ff4d2b869a1ee6b2e2ca31d66ec4bbde4287f5a777838ffe932cd15b993cb0224b86e43d684de61c35acbcd0 (sha512sum)
1.9.5 Packages (March 31st 2019)
Source
- pygame-1.9.5.tar.gz ~ 3.1M ~ 72bec05e052f1b271f4fab219d078d0f768a72ea (sha1)
1.9.4.post1 Packages (Oct 27th 2018)
Source
- pygame-1.9.4.post1.tar.gz ~ 2.9M ~ 956e43144348d9a05a40d5a381b5eaee
1.9.4 Packages (July 19th 2018)
Source
- pygame-1.9.4.tar.gz ~ 4.6M ~ 9387835fab92a8b4a3c9e51e2c9267a670476aaa
Wheel packages are also available on PyPI, and may be installed by running pip install wheel
1.9.3 Packages (January 16th 2017)
Source
- pygame-1.9.3.tar.gz ~ 2M
Wheel packages are also available on PyPI, and may be installed by running pip install wheel
1.9.1 Packages (August 6th 2009)
Source
- pygame-1.9.1release.tar.gz ~ 1.4M - source/docs/examples in unix format
- pygame-1.9.1release.zip ~ 1.5M - source/docs/examples in windows format
Windows
Visual Studio Code Python
Get the version of pygame for your version of python. You may need to uninstall old versions of pygame first.NOTE: if you had pygame 1.7.1 installed already, please uninstall it first. Either using the uninstall feature - or remove the files: c:python25libsite-packagespygame . We changed the type of installer, and there will be issues if you don't uninstall pygame 1.7.1 first (and all old versions).
- pygame-1.9.1.win32-py2.7.msi 3.1MB
- pygame-1.9.1release.win32-py2.4.exe 3MB
- pygame-1.9.1release.win32-py2.5.exe 3MB
- pygame-1.9.1.win32-py2.5.msi 3MB
- pygame-1.9.1.win32-py2.6.msi 3MB
- pygame-1.9.2a0.win32-py2.7.msi 6.4MB
- pygame-1.9.1.win32-py3.1.msi 3MB
- pygame-1.9.2a0.win32-py3.2.msi 6.4MB
- (optional) Numeric for windows python2.5 (note: Numeric is old, best to use numpy) http://rene.f0o.com/~rene/stuff/Numeric-24.2.win32-py2.5.exe
- windows 64bit users note: use the 32bit python with this 32bit pygame.
Unix Distributions
1.9.1 has been packaged up for almost all major distributions. You can also install 1.9.1 from source with python setup.py install (see Compilation page).- Ubuntu - pygame is available through apt-get in the Universe (1.9.1)
- Gentoo - pygame is available in the portage system (1.9.1 + 1.9.2prerelease)
- Fedora - Package repositories have support for pygame (1.9.1)
- Suse - The Yast package system has pygame (updated to 1.9.1)
- OLPC - comes with the XO, and sugar (1.9.1).
- archlinux - pygame is available through pacman (1.9.1)
- Debian - pygame is available through apt-get (1.9.1)
- FreeBSD - pygame is included in standard ports as py26-game (1.8.1)
- OpenBSD - pygame is included in standard ports (1.8.1)
Macintosh

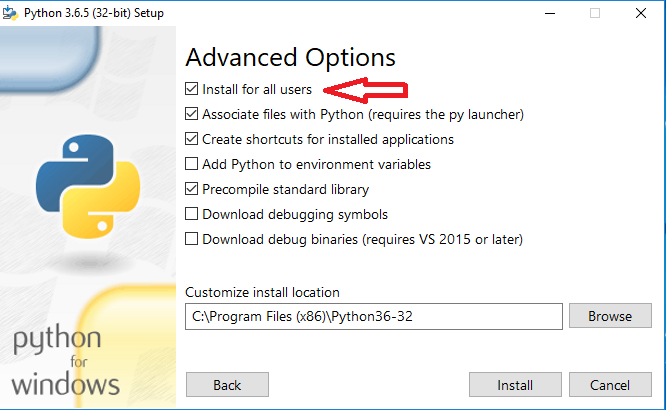
- pygame-1.9.1release-python.org-32bit-py2.7-macosx10.3.dmg 12MB
- pygame-1.9.1release-py2.6-macosx10.5.zip 10.3MB
- pygame-1.9.1release-py2.5-macosx10.5.zip 10.3MB
- pygame-1.9.1release-py2.4-macosx10.5.zip 10.3MB
- MacPorts - available in the ports collection as py-game (updated to 1.9.1)
- fink - 1.7.1release is available. (no bug submitted yet for 1.9.1 update)
- Snow leopard osx apple supplied python: pygame-1.9.2pre-py2.6-macosx10.6.mpkg.zip
- Lion apple supplied python: pygame-1.9.2pre-py2.7-macosx10.7.mpkg.zip
Nokia
- nokia pys60 - pygame-S60-1.9.0_pyS60-1.9.7_SVN-2559_20090805_GCCE-UREL.sisx
- Maemo - latest release version 1.8.1 - in package manager. (bug report for 1.9.1 update)
BeOS
- Haiku-os - (an open source BeOS) latest release version 1.8.1 (no bug submitted yet for 1.9.1 update)
- Bebits - latest release version 1.6
Android
Other
- prebuilt-msvcr71.zip ~ 1.7M - March 29th 2008 - all win32 dependency libraries.
- Pygame-1.8.0-deps-src.zip ~ 19.7M - June 29 2008 - all win32 source dependencies.
- pygame2exe.py ~ 1 kb - Nov 11, 2002 - run py2exe on your pygames.
- Aliens-0.9-win32.exe ~ 1.3 mb - Standalone Aliens example for windows.
- pygame_logo.psd ~ 1.3 mb - Highres version of the logo, in photoshop format with layers.
- pyobjc-1.4-py2.5-macosx10.4.mpkg.zip - pyobjc is needed for old versions of pygame1.8.x on OSX 10.3, 10.4, and 10.5.
Previous Releases
Vs Code Pygame
- pygame-1.9.0release.tar.gz ~ 1.4M - August 1, 2009
- pygame-1.8.1release.tar.gz ~ 1.4M - July 30, 2008
- pygame-1.8.0release.tar.gz ~ 1.4M - March 29, 2008
- pygame-1.7.1release.tar.gz ~ 1.3M - August 16, 2005
- 1.7.0 ~ no source release was made.
- pygame-1.6.2.tar.bz2 ~ 1140 kb -
- pygame-1.6.tar.gz ~ 832 kb - October 23, 2003
- pygame-1.5.tar.gz ~ 736 kb - May 30, 2002
- pygame-1.4.tar.gz ~ 808 kb - Jan 30, 2002
- pygame-1.3.tar.gz ~ 731 kb - Dec 19, 2001
- pygame-1.2.tar.gz ~ 708 kb - Sep 4, 2001
- pygame-1.1.tar.gz ~ 644 kb - Jun 23, 2001
- pygame-1.0.tar.gz ~ 564 kb - Apr 5, 2001
- pygame-0.9.tar.gz ~ 452 kb - Feb 13, 2001
- pygame-0.5.tar.gz ~ 436 kb - Jan 6 14, 2001
- pygame-0.4.tar.gz ~ 420 kb - Dec 14, 2000
- pygame-0.3b.tar.gz ~ 367 kb - Nov 20, 2000
- pygame-0.2b.tar.gz ~ 408 kb - Nov 3, 2000
- pygame-0.1a.tar.gz ~ 300 kb - Oct 28, 2000
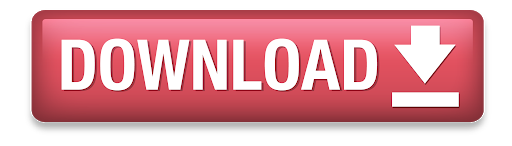